Webhook events
Webhook authentication
We've taken some steps to secure webhook communications, so you can always verify that a webhook event came from us and not from a malicious party.
Below are the steps you need to follow to verify that a webhook event came from Bridgecard.
STEP 1: Access your webhook secret from your dashboard (live_webhook_secret_key or test_webhook_secret_key depending on the environment)
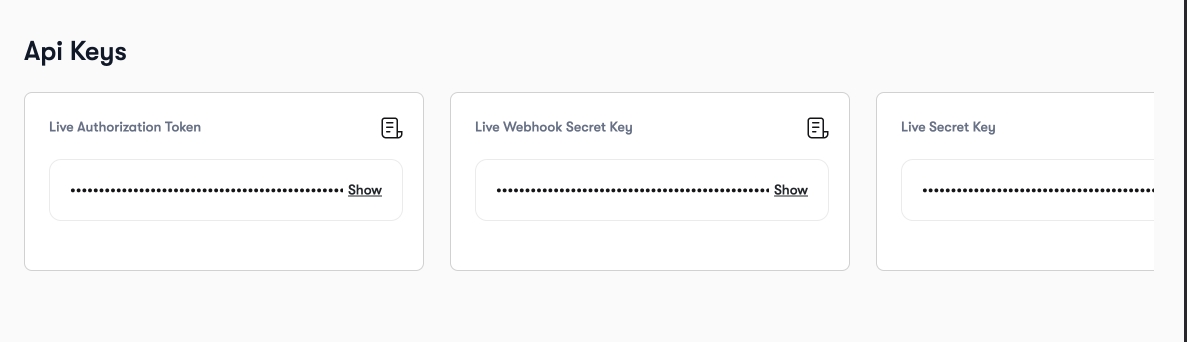
STEP 2: Whenever you get a webhook event from Bridgecard, extract the x-webhook-signature header and decrypt it using your secret key (live_secret_key / test_secret_key depending on the environment)
$ pip install aes-everywhere
from AesEverywhere import aes256
# decryption
decrypted = aes256.decrypt('x-webhook-signature value', 'Bridgecard Secret Key')
decrypted = decrypted.decode()
print(decrypted)
STEP 3: Compare the decrypted string with your webhook secret key from STEP 1. If they are the same, then it is a valid webhook event sent from us.
All webhook events
These are the list of webhook events triggered when you communicate with our APIs.
cardholder_verification.successful
This webhook event is sent when a user has passed the ID verification process
{
"event": "cardholder_verification.successful",
"data" : {
"cardholder_id": "859505050505",
"is_active": true,
"livemode": false,
"issuing_app_id": "9ujinoncpsni3943198393939930ke"
}
}
cardholder_verification.failed
This webhook event is sent when a user has failed the ID verification process
{
"event": "cardholder_verification.failed",
"data" : {
"cardholder_id": "859505050505",
"is_active": true,
"livemode": false,
"issuing_app_id": "9ujinoncpsni3943198393939930ke",
"error_description": "the user passed an invalid ID number"
}
}
card_creation_event.successful
We send this webhook event when a card is successfully created
{
"event": "card_creation_event.successful",
"data" : {
"card_id": "859505050505",
"cardholder_id": "859505050505",
"currency": "USD",
"issuing_app_id": "9ujinoncpsni3943198393939930ke",
"livemode": True
"meta_data": {"user_id": "d0658fedf828420786e4a7083fa"}
}
}
card_creation_event.failed
This webhook event is sent when we can't complete your request to create a card.
{
"event": "card_creation_event.failed",
"data" : {
"card_id": "859505050505",
"cardholder_id": "859505050505",
"currency": "USD",
"issuing_app_id": "9ujinoncpsni3943198393939930ke",
"livemode": True,
"reason": "The user failed our second stage KYC verification for fraud detection, please contact admin."
}
}
card_credit_event.successful
We send this webhook event when a successful credit transaction happens on a USD card.
{
"event": "card_credit_event.successful",
"data" : {
"card_id": "859505050505",
"cardholder_id": "859505050505",
"amount": "100",
"currency": "USD",
"transaction_reference":"859505050505",
"livemode": false,
"issuing_app_id": "9ujinoncpsni3943198393939930ke",
"card_transaction_type": "CREDIT",
"transaction_date": "2022-09-28 23:10:45",
"transaction_timestamp": "1664406645",
"settled_available_balance":"500",
"settled_book_balance":"400"
}
}
card_credit_event.failed
We send this webhook event when a card funding transaction fails on a USD card.
{
"event": "card_credit_event.failed",
"data" : {
"card_id": "859505050505",
"cardholder_id": "859505050505",
"amount": "100",
"currency": "USD",
"transaction_reference":"859505050505",
"livemode": false,
"issuing_app_id": "9ujinoncpsni3943198393939930ke",
"card_transaction_type": "CREDIT",
"transaction_date": "2022-09-28 23:10:45",
"transaction_timestamp": "1664406645"
}
}
card_unload_event.successful
You get this webhook event when an unload transaction is successful on a USD card.
{
"event": "card_unload_event.successful",
"data" : {
"card_id": "859505050505",
"cardholder_id": "859505050505",
"amount": "100",
"description": "Virtual dollar card unloading",
"currency": "USD",
"transaction_reference":"859505050505",
"livemode": false,
"issuing_app_id": "9ujinoncpsni3943198393939930ke",
"card_transaction_type": "DEBIT",
"transaction_date": "2022-09-28 23:10:45",
"transaction_timestamp": "1664406645"
}
}
card_unload_event.failed
You get this webhook event when an unload transaction on a USD card fails.
{
"event": "card_unload_event.failed",
"data" : {
"card_id": "859505050505",
"cardholder_id": "859505050505",
"amount": "100",
"description": "Virtual dollar card unloading",
"currency": "USD",
"transaction_reference":"859505050505",
"livemode": false,
"issuing_app_id": "9ujinoncpsni3943198393939930ke",
"card_transaction_type": "DEBIT",
"transaction_date": "2022-09-28 23:10:45",
"transaction_timestamp": "1664406645"
}
}
card_debit_event.successful
You get this webhook event when a debit transaction happens on a USD card.
{
"event": "card_debit_event.successful",
"data" : {
"card_id": "859505050505",
"cardholder_id": "859505050505",
"amount": "100",
"description": "Amazon US",
"currency": "USD",
"transaction_reference":"859505050505",
"livemode": false,
"issuing_app_id": "9ujinoncpsni3943198393939930ke",
"card_transaction_type": "DEBIT",
"merchant_category_code": "12345 can be None",
"transaction_date": "2022-09-28 23:10:45",
"transaction_timestamp": "1664406645",
"settled_available_balance":"500",
"settled_book_balance":"400"
}
}
card_debit_event.declined
We send this webhook event whenever there's a declined transaction on the user's USD card.
{
"data": {
"amount": "100",
"card_id": "06246484994004040404efcf",
"cardholder_id": "06246484994004040404efcf",
"currency": "USD",
"decline_reason": "Transaction is not permitted. Please confirm that the billing address you're using is 256 Chapman Road STE 105-4, Newark, 19702, Delaware (DE) and try again. Also confirm from support if our cards work on this platform.",
"description": "APPLE ECOM FBILL APPLE ECOM FBILL 866 D712 D7753",
"issuing_app_id": "5b29cbe5b29cbe5b29cbe5b29cbe5b29cbe",
"livemode": true,
"transaction_date": "2024-02-27 19:54:51",
"transaction_reference": "3cdee95e5e0c883cdee95e5e0c883cdee95e5e0c883cdee95e5e0c88",
"transaction_timestamp": "1709063691"
},
"event": "card_debit_event.declined"
}
card_declined_transaction_fee_charge_event.successful(Deprecated)
We send this webhook event when the declined transaction fee on a user's card has been charged.
{
'event': 'card_declined_transaction_fee_charge_event.successful,
'data': {
'card_id': '8eedfssfee443rffd1cb5e',
'cardholder_id': 'bfgg8eedfssfee443rffd1cb5e',
'currency': 'USD',
'amount': '30',
'transaction_date': '2021-11-15 17:13:19',
'transaction_timestamp': '1636996399',
'transaction_reference': '859505050505',
'livemode': True,
'issuing_app_id': 'c6424f3c-1708-4386-9406-b20da7f4d6b9',
'transaction_date': '2022-09-28 23:10:45',
'transaction_timestamp': '1664406645',
'card_transaction_type': 'DEBIT'
}
}
card_reversal_event.successful
This webhook event is triggered whenever a user USD card gets a reversal from a merchant that they previously got debited from.
{
"event": "card_reversal_event.successful",
"data": {
"card_id": "a303fb8b7a0e476fbc910671ca952b74",
"cardholder_id": "9b78a44ccdf848fd860f75fd5c117d9d",
"amount": "100",
"currency": "USD",
"transaction_reference": "22597F3D-A7A5-4C9B-B9DF-B7D918EE8241_REVERSAL",
"transaction_date": "2022-09-28 23:10:45",
"transaction_timestamp": "1664406645",
"livemode": true,
"issuing_app_id": "c6424f3c-1708-4386-9406-b20da7f4d6b9",
"card_transaction_type": "CREDIT"
}
}
card_delete_event.notification
This webhook event is triggered to notify a user that he doesn't have enough funds to pay the maintenance fee due in the subsequent month and his card will be automatically deleted in some days.
{
"event": "card_delete_event.notification",
"data" : {
"card_id": "859505050505",
"cardholder_id": "859505050505",
"currency": "USD",
"issuing_app_id": "9ujinoncpsni3943198393939930ke",
"potential_delete_date": "2022-09-28 23:10:45",
"livemode": True
}
}
card_delete_event.successful
This webhook event is triggered whenever a user's USD card gets deleted. Note: USD Mastercard gets deleted if a user doesn't have enough balance to pay for the next month's monthly maintenance fee.
{
"event": "card_delete_event.successful",
"data" : {
"card_id": "859505050505",
"cardholder_id": "859505050505",
"currency": "USD",
"issuing_app_id": "9ujinoncpsni3943198393939930ke",
"livemode": True,
"balance": "400",
"reason": "" // can be None.
}
}
3d_secure_otp_event.generated [Deprecated]
This webhook event is sent when a user attempts a transaction online and he's sent an OTP to confirm the transaction.
{
"event": "3d_secure_otp_event.generated",
"data": {
"card_id": "86a19202202002020251i292",
"cardholder_id": "86a19202202002020251i292",
"otp": "430854",
"merchant_name": "BRIDGECARD",
"amount": "300",
"currency": "USD",
"last_four_digits": "4804",
"issuing_app_id": "198286a192020251i292b01ab",
"livemode": true,
"transaction_date": "2023-02-02 23:49:00",
"transaction_timestamp": "1675381740"
}
}
Note:
This API is currently deprecated. To get OTP for transactions on the Mastercard kindly call the transaction history API to fetch the OTP for that current transaction.
Here is a sample. In this example, the OTP required for FACEBOOK is CB966S3VM2
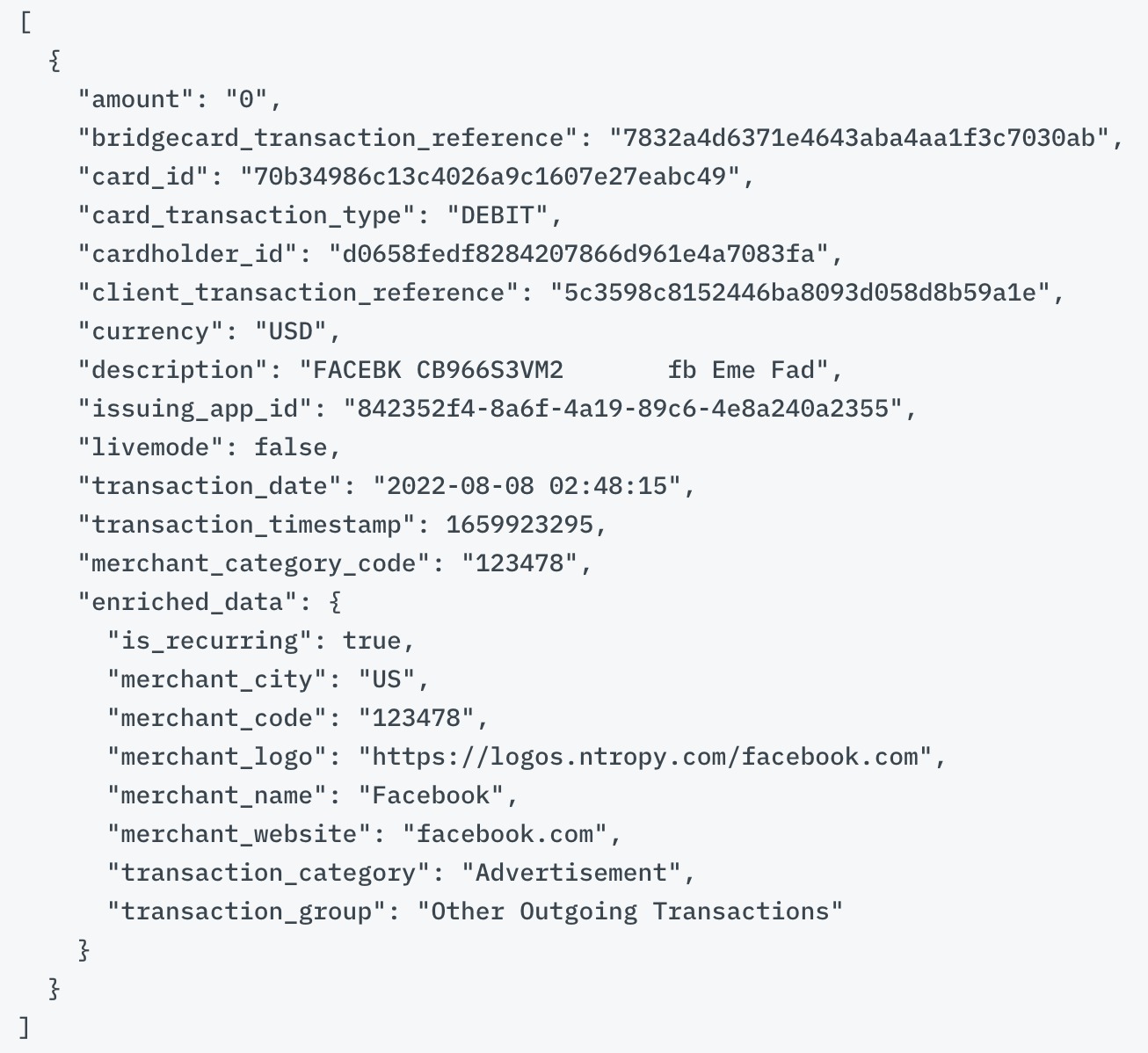
card_maintenance_fee_debit_event.successful
We send this webhook event whenever when we deduct the monthly card maintenance fee from the card.
{
"event": "card_maintenance_fee_debit_event.successful",
"data": {
"card_id": "38f155a93148",
"cardholder_id": "08ceb6a9",
"amount": "100",
"currency": "USD",
"transaction_date": "2023-04-30 11:21:18",
"transaction_timestamp": "1682853678",
"livemode": true,
"issuing_app_id": "e9ab",
"card_transaction_type": "DEBIT"
}
}
card_freezed_due_to_30_days_inactivity_event.successful
This webhook event is sent when a user's card has been blocked because he hasn't done any transactions in the last 30 days.
{
"event": "card_freezed_due_to_30_days_inactivity_event.successful",
"data": {
"card_id": "38f155a9314838f155a93148",
"cardholder_id": "08ceb6a908ceb6a908ceb6a9",
"livemode": true,
"issuing_app_id": "e9ab08ceb6a908ceb6a908ceb6a9",
"block_reason": "The user has not made any transactions in the last 30 days."
}
}
card_flagged_due_to_suspiscion_of_fraud.activated
This webhook event is sent when a user's card has been blocked due to suspicion of fraudulent activity. We'll have to manually review this case and make a final decision, you can reach out to support in this case.
{
"event": "card_flagged_due_to_suspiscion_of_fraud.activated",
"data": {
"card_id": "38f155a9314838f155a93148",
"cardholder_id": "08ceb6a908ceb6a908ceb6a9",
"livemode": true,
"issuing_app_id": "e9ab08ceb6a908ceb6a908ceb6a9",
"reason": "This card has been flagged due to suspicion of fraudulent transactions."
}
}
naira_card_credit_event.successful
We send this webhook event when a successful credit transaction happens on a Naira card.
{
"event":"naira_card_credit_event.successful",
"data":{
"card_id":"1104776035",
"transaction_reference":"190220249992843051",
"cardholder_id":"016ff9d57b314d57aab3cfaf22f53840",
"currency":"NGN",
"issuing_app_id":"e98260dc-747f-4fa8-8b6c-4704a89b01ab",
"livemode":false,
"amount":"500",
"transaction_reference": "57b314d57aab3016ff9d57b314d57aa",
"transaction_type":"CREDIT",
"transaction_date": "2022-09-28 23:10:45",
"transaction_timestamp": "1664406645"
}
}
naira_card_credit_event.failed
We send this webhook event when a credit transaction fails on a Naira card.
{
"event":"naira_card_credit_event.failed",
"data":{
"card_id":"1104776035",
"transaction_reference":"190220249992843051",
"cardholder_id":"016ff9d57b314d57aab3cfaf22f53840",
"currency":"NGN",
"issuing_app_id":"e98260dc-747f-4fa8-8b6c-4704a89b01ab",
"livemode":false,
"amount":"500",
"transaction_reference": "57b314d57aab3016ff9d57b314d57aa",
"transaction_type":"CREDIT",
"transaction_date": "2022-09-28 23:10:45",
"transaction_timestamp": "1664406645"
}
}
naira_card_unload_event.successful
You get this webhook event when an unload transaction is successful on a Naira card.
{
"event":"naira_card_unload_event.successful",
"data":{
"card_id":"1104776035",
"transaction_reference":"190220249992843051",
"cardholder_id":"016ff9d57b314d57aab3cfaf22f53840",
"currency":"NGN",
"issuing_app_id":"e98260dc-747f-4fa8-8b6c-4704a89b01ab",
"livemode":false,
"amount":"500",
"transaction_reference": "57b314d57aab3016ff9d57b314d57aa",
"transaction_type":"DEBIT",
"transaction_date": "2022-09-28 23:10:45",
"transaction_timestamp": "1664406645"
}
}
naira_card_unload_event.failed
You get this webhook event when an unload transaction failed on a Naira card.
{
"event":"naira_card_unload_event.failed",
"data":{
"card_id":"1104776035",
"transaction_reference":"190220249992843051",
"cardholder_id":"016ff9d57b314d57aab3cfaf22f53840",
"currency":"NGN",
"issuing_app_id":"e98260dc-747f-4fa8-8b6c-4704a89b01ab",
"livemode":false,
"amount":"500",
"transaction_reference": "57b314d57aab3016ff9d57b314d57aa",
"transaction_type":"DEBIT",
"transaction_date": "2022-09-28 23:10:45",
"transaction_timestamp": "1664406645"
}
}
naira_card_debit_event.successful
You get this webhook event when an debit transaction is successful on a Naira card.
{
"event":"naira_card_debit_event.successful",
"data":{
"cardholder_id":"30429e8efce74ad3a0b2df8bf9189136",
"amount":"57272",
"currency":"NGN",
"is_successful":true,
"transaction_type":"DEBIT",
"transaction_reference":"0603202499930495",
"issuing_app_id":"ef4ad945-e99b-4507-8609-709b144f77d4",
"livemode":false,
"description":"POINT OF SALE PURCHASE TRANSACTION POS@<99999999> <284118000200925> <APPLE.COM/BILL \\xa0 \\xa0 \\xa0 \\xa0 ITUNES.COM \\xa0 \\xa0 IE> <520360/129268> ",
"transaction_timestamp":1709688060,
"is_card":true,
"transaction_date":"2024-03-06 02:21:00",
"enriched_transaction":{
"transaction_category":"Media",
"transaction_group":"Non-Essential Expenses",
"merchant_name":"Apple",
"merchant_logo":"https://logos.ntropy.com/apple.com",
"merchant_website":"apple.com",
"merchant_city":"None",
"is_recurring":true,
"merchant_code":"None"
}
}
}
naira_card_debit_event.declined
You get this webhook event when a debit transaction is declined on a Naira card.
{
"event":"naira_card_debit_event.declined",
"data":{
"cardholder_id":"30429e8efce74ad3a0b2df8bf9189136",
"amount":"57272",
"currency":"NGN",
"is_successful":true,
"transaction_type":"DEBIT",
"transaction_reference":"0603202499930495",
"issuing_app_id":"ef4ad945-e99b-4507-8609-709b144f77d4",
"livemode":false,
"description":"POINT OF SALE PURCHASE TRANSACTION POS@<99999999> <284118000200925> <APPLE.COM/BILL \\xa0 \\xa0 \\xa0 \\xa0 ITUNES.COM \\xa0 \\xa0 IE> <520360/129268> ",
"transaction_timestamp":1709688060,
"is_card":true,
"transaction_date":"2024-03-06 02:21:00",
"enriched_transaction":{
"transaction_category":"Media",
"transaction_group":"Non-Essential Expenses",
"merchant_name":"Apple",
"merchant_logo":"https://logos.ntropy.com/apple.com",
"merchant_website":"apple.com",
"merchant_city":"None",
"is_recurring":true,
"merchant_code":"None"
}
}
}
naira_account_credit_event.successful
This webhook event is sent when a user's naira account is credited
{
'event': 'naira_account_credit_event.successful',
'data': {
'transaction_reference': '100720239194959',
'cardholder_id': '019349be694f4daea1a9b04c0',
'currency': 'NGN',
'issuing_app_id': 'e98260dc-sdfdsf-wewcw2-8b6c-4704a89b01ab',
'livemode': False,
'amount': '995000',
'description': 'VPS TRANSFERS TRANSFER FROM JOHN DOE TO BRIDGECARD(Bridgecard) 99**92 Wallet fund ',
'transaction_type': 'CREDIT'
}
}
naira_account_transfer_event.successful
This webhook event is sent when a user's naira account debit transaction is successful
{
"event":"naira_account_transfer_event.successful",
"data":{
"cardholder_id": "48499494",
"amount": "995000",
"currency":"NGN",
"is_successful": true,
"transaction_type": "DEBIT",
"recipient_account_number":"5403200440",
"recipient_bank_code":"0184",
"transaction_reference":"100343243439991194959",
"livemode": true,
"issuing_app_id":"e982-4fa8-8b6c-47b01ab",
}
}
naira_account_transfer_event.failed
This webhook event is sent when a user's naira account debit transaction fails
{
"event":"naira_account_transfer_event.failed",
"data":{
"cardholder_id": "48499494",
"amount": "995000",
"currency":"NGN",
"is_successful": true,
"transaction_type": "DEBIT",
"recipient_account_number":"5403200440",
"recipient_bank_code":"0184",
"transaction_reference":"100343243439991194959",
"livemode": true,
"issuing_app_id":"e982-4fa8-8b6c-47b01ab",
}
}
rewards_event.claimed
This webhook event is sent when a user claims a reward via the rewards service.
{
"event": "rewards_event.claimed",
"data": {
"coupon_code": "25FY1SL4O9DI",
"created_on": "19/08/2023",
"description": "Women Leggings Black White",
"downvotes": 0,
"is_available": true,
"merchant": "Aliexpress",
"reward_id": "JDDDNDNDNDND",
"reward_type": "PROMO_CODE",
"success_record": 0,
"upvotes": 0,
"valid_till": 1694818799,
"valid_till_date": "15/09/2023",
"cardholder_id": "48499494",
},
}
card_migration_event.successful (Deprecated)
We send this webhook event when a card is successfully migrated
{
"event": "card_migration_event.successful",
"data" : {
"card_id": "859505050505",
"cardholder_id": "859505050505",
"currency": "USD",
"issuing_app_id": "9ujinoncpsni3943198393939930ke",
"livemode": True
}
}
cardholder_verification.manual_review
This webhook event is sent when a cardholder KYC fails and is subject to manual review within 24 hours.
{
"event": "cardholder_verification.manual_review",
"data" : {
"cardholder_id": "859505050505",
"is_active": False,
"issuing_app_id": "9ujinoncpsni3943198393939930ke",
"livemode": True,
}
}
card_migration_event.failed (Deprecated)
This webhook event is sent when we can't complete your request to migrate a card.
{
"event": "card_creation_event.failed",
"data" : {
"card_id": "859505050505",
"cardholder_id": "859505050505",
"currency": "USD",
"issuing_app_id": "9ujinoncpsni3943198393939930ke",
"livemode": True,
"reason": "You don't have sufficient funds to create a card with this limit, you need to fund with up to $3 to create a card with a $5,000 limit and up to $4 for a card with $10,000 limit."
}
}
Issuing_account_topup.successful
This webhook is sent after a successful top-up on your issuing wallet.
{
"environment": “production”,
"issuing_app_id": “9ujinoncpsni3943198393939930ke”,
"data": {
"event": "issuing_account_topup.successful",
"data": {
"amount_topped_up_in_cents": 200
},
},
}
card_negative_balance_event.notification
This webhook is sent when a card recieves a transaction update and the card balance goes into negative. Imagine your card has only a $10 balance. If you book an Uber ride with an estimated cost of $10, the ride cost is authorized and debited from your card right away. However, if an unforeseen event, like an accident, extends the ride and the cost increases to $15, the final amount will be adjusted during the settlement stage (typically within 0-3 days). In this case, the transaction amount will update to $15, resulting in a $5 negative balance on your card. Uber reserves the right to apply this negative balance to the card.
{
"event": "card_negative_balance_event.notification",
"data": {
"card_id": "637387383939ee3003838033",
"cardholder_id": "637387383939ee3003838033",
"currency": "USD",
"issuing_app_id": "637387383939ee3003838033",
"livemode": true,
"merchant_name": "FACEBK *HDB9XEQ9S2 Dublin "
}
}
Last updated